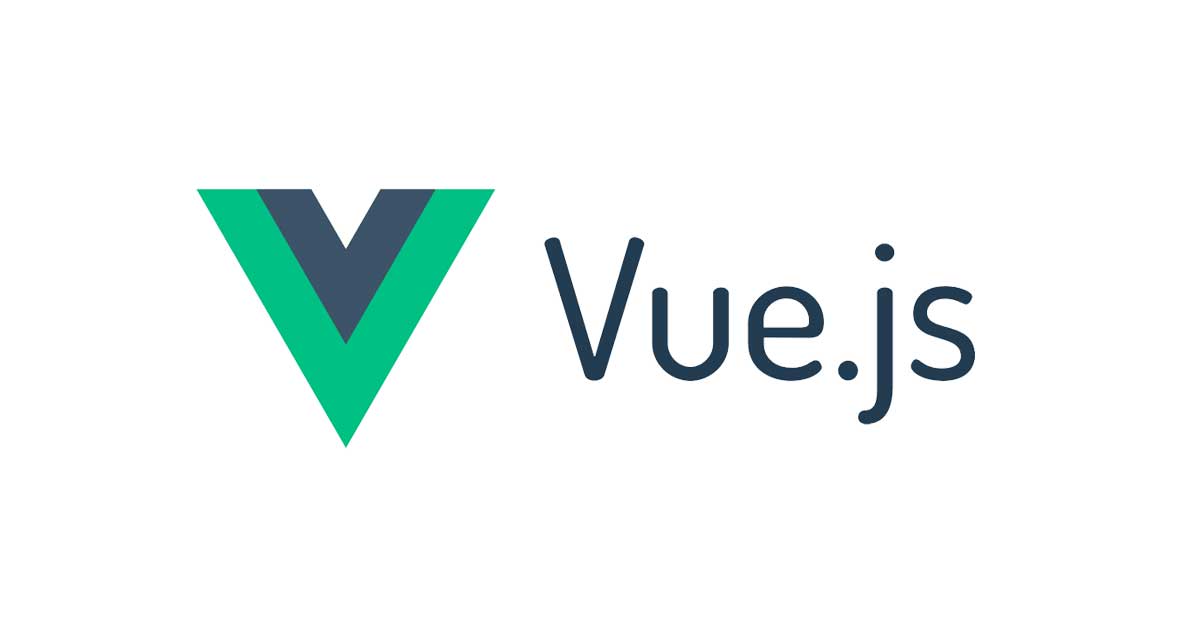
Nuxt.jsでTypeScriptを使う(with vue-property-decorator)
概要
スクラッチでNuxt.jsをTypeScriptを利用して開発する環境作成の手順を確認したかったので、方法を調べつつ実際に作成してみました。
できる限りミニマムな構成で作成したいのと、自分的には理解のブラックボックス化が進みやすいため可能な限りVue CLIやcreate-nuxt-appは使用したくなかったため使用していません。(より簡単に早く、動くものを作りたい場合にはVue CLIやcreate-nuxt-appの利用をおすすめします。)
前提
- Nuxt.js 2.14.4を利用
- html-property-decoratorを利用
やってみたこと
以下の流れで、index.vueに内容されている子コンポーネントに値を引き渡して表示するアプリケーションを作成してみました。
- 必要なライブラリのインストール
- nuxt, nuxt/typescript-build等のインストール
- package.json、nuxt.config.js、tsconfig.jsonの設定
- コンテンツの作成
- index.vueページと子コンポーネントの作成
- 動作確認
- nuxtコマンドによる動作確認
1. 必要なライブラリのインストール
$ vim package.json $ mkdir pages $ vim pages/index.vue $ npm i --save nuxt $ npm i --save-dev @nuxt/typescript-build @nuxt/types $ npm i --save vue-property-decorator $ npm i --save vue-class-component $ vim nuxt.config.js $ vim tsconfig.json
package.json
{ "name":"app", "scripts":{ "dev":"nuxt" } }
index.vue
<template> <h1>This is test.</h1> </template>
nuxt.config.js
export default { buildModules: ['@nuxt/typescript-build'] }
tsconfig.json
{ "compilerOptions": { "experimentalDecorators": true, "target": "ES2018", "module": "ESNext", "moduleResolution": "Node", "lib": [ "ESNext", "ESNext.AsyncIterable", "DOM" ], "esModuleInterop": true, "allowJs": true, "sourceMap": true, "strict": true, "noEmit": true, "baseUrl": ".", "paths": { "~/*": [ "./*" ], "@/*": [ "./*" ] }, "types": [ "@types/node", "@nuxt/types" ] }, "exclude": [ "node_modules" ], }
2. コンテンツの作成
- コンポーネントを作成
$ mkdir components $ vim components/TestComponent.vue
components/TestComponent.vue
<template> <div> Name: {{ fullName }} Message: {{ message }} </div> </template> <script lang="ts"> import { Vue, Component, Prop } from 'vue-property-decorator' interface User { firstName: string lastName: string } @Component export default class TestComponent extends Vue { @Prop({ type: Object, required: true }) readonly user!: User message: string = 'This is a message' get fullName (): string { return `${this.user.firstName} ${this.user.lastName}` } } </script>
- 作成したコンポーネントに合わせてindex.vueを編集
$ vim pages/index.vue
index.vue
<template> <div> <h1>This is Test</h1> <TestComponent :user="testUser" /> </div> </template> <script lang="ts"> import { Component, Vue } from "vue-property-decorator"; import TestComponent from "~/components/TestComponent.vue"; @Component({ components: { TestComponent, }, }) export default class IndexPage extends Vue { myname = { firstName: "aki", lastName: "kato" } get testUser(){ return this.myname } } </script>
3. 動作確認
- index.vueでTestComponentに渡したプロパティをもとに、コンポーネント側で表示を行うことができたことを確認します
- 正常に表示されていれば、TestConponent.vueでは
data()
やcomputed:{}
等の記述の代わりに、TypeScriptによる記述で同じことを実現できたことが確認できます
参考
この記事を書くにあたって参考にさせていただいたサイトは下記になります
- Nuxt TypeScript https://typescript.nuxtjs.org/ja/
おわりに
vue-property-decoratorを利用してNuxt.jsでTypeScriptを使うためのミニマムな動作サンプルを作成してみた内容の紹介でした。
どなたかの参考になれば幸いです。